728x90
GridControl 에 데이터 바인딩시 특정 컬럼을 색으로 표시하는 방법입니다.
xaml 코드에 아래 처럼 Colum을 정의하고 Data 로 접근해 Brush Type 필드를 지정합니다.
<dxg:GridColumn FieldName="CellColor" Header="COLOR" HorizontalHeaderContentAlignment="Center"> <dxg:GridColumn.CellTemplate> <DataTemplate> <Border Background="{Binding Data.CellColor}" /> </DataTemplate> </dxg:GridColumn.CellTemplate> </dxg:GridColumn> |
ItemModel.cs
using System.Windows.Media;
namespace Wpf.DevGridTest
{
public class ItemModel
{
public Brush CellColor { get; set; }
public string Name { get; set; }
public int Age { get; set; }
}
}
MainWindow.xaml
xmlns:dxg="http://schemas.devexpress.com/winfx/2008/xaml/grid"
<dxg:GridControl
Grid.Row="3"
Height="300"
ItemsSource="{Binding Items}">
<dxg:GridColumn
FieldName="CellColor"
Header="COLOR"
HorizontalHeaderContentAlignment="Center">
<dxg:GridColumn.CellTemplate>
<DataTemplate>
<Border Background="{Binding Data.CellColor}" />
</DataTemplate>
</dxg:GridColumn.CellTemplate>
</dxg:GridColumn>
<dxg:GridColumn
FieldName="Name"
Header="NAME"
HorizontalHeaderContentAlignment="Center" />
<dxg:GridColumn
FieldName="Age"
Header="AGE"
HorizontalHeaderContentAlignment="Center" />
<dxg:GridControl.View>
<dxg:TableView
CellStyle="{StaticResource FocusedCellStyle}"
RowStyle="{StaticResource FocusedRowStyle}"
ShowGroupPanel="False"
ShowGridMenu="TableView_ShowGridMenu"/>
</dxg:GridControl.View>
</dxg:GridControl>
MainViewModel.cs
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.ComponentModel;
using System.Dynamic;
using System.Runtime.CompilerServices;
using System.Windows.Media;
namespace Wpf.DevGridTest
{
public class MainViewModel : INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
public void OnPropertyChanged([CallerMemberName] string name = "") => PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(name));
private ObservableCollection<ItemModel> items;
public ObservableCollection<ItemModel> Items
{
get
{
if (this.items == null)
{
this.items = new ObservableCollection<ItemModel>();
}
return this.items;
}
set
{
this.items = value;
OnPropertyChanged();
}
}
public MainViewModel()
{
// Cell Color
this.Items.Add(new ItemModel() { CellColor = new SolidColorBrush(System.Windows.Media.Colors.Blue), Name = "홍길동", Age = 45 });
this.Items.Add(new ItemModel() { CellColor = new SolidColorBrush(System.Windows.Media.Colors.Yellow), Name = "유관순", Age = 57 });
this.Items.Add(new ItemModel() { CellColor = new SolidColorBrush(System.Windows.Media.Colors.Green), Name = "이순신", Age = 60 });
this.Items.Add(new ItemModel() { CellColor = new SolidColorBrush(System.Windows.Media.Colors.Red), Name = "강감찬", Age = 22 });
this.Items.Add(new ItemModel() { CellColor = new SolidColorBrush(System.Windows.Media.Colors.Purple), Name = "윤봉길", Age = 15 });
}
}
}
결과
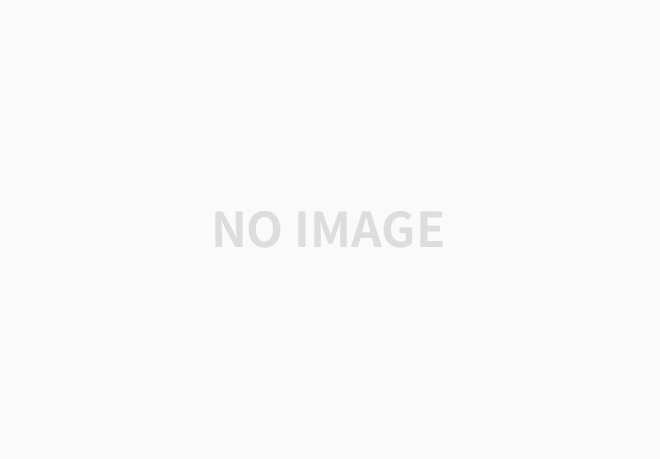
소스
https://github.com/kei-soft/KJunBlog/tree/master/Wpf.DevGridTest
728x90
'DevExpress' 카테고리의 다른 글
[DevExpress/WPF] GridControl Contextmenu 표시하기 (0) | 2022.09.16 |
---|---|
[DevExpress/WPF] GridControl Cell 선택되지 않게 하기 (CanSelectCell) (0) | 2022.09.15 |
[DevExpress/WPF] GridControl ReadOnly 처리하기 (0) | 2022.09.15 |
[DevExpress/WPF] GridControl 에 ExpandObject 바인딩 하기 (0) | 2022.09.15 |
[DevExpress/WPF] DateEdit 기간으로 표시하기 (-10 ~ 10) (0) | 2022.09.02 |